Hello friends, in this tutorial we will develop a custom client side people picker control in SharePoint. It is applicable for SharePoint 2013, 2016 and SharePoint Online.
In the first section, we will save the people picker value in SharePoint list (people picker field) using REST API.
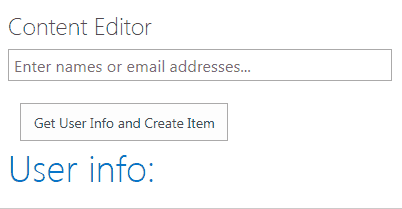
In the second section we will see, how to get the value of people picker field from SharePoint list and set to our custom people picker field, as shown below:
Section 1: Create custom people picker field and save its value in SharePoint list.
Step 1: Create a list named EmpDetails. Create a person or group field named EmployeeName. We are going to save of custom field value in this list field.
Step 2: Create a webpart page and add content editor webpart
Step 3: Add below code and we are ready with our custom field.
Download below code: Download link
<html> <head> <script src="/teams/SPOSupportSite/CustomCode/jquery.min.js"></script> <script src="/_layouts/15/sp.runtime.js"></script> <script src="/_layouts/15/sp.js"></script> <script src="/_layouts/15/1033/strings.js"></script> <script src="/_layouts/15/clienttemplates.js"></script> <script src="/_layouts/15/clientforms.js"></script> <script src="/_layouts/15/clientpeoplepicker.js"></script> <script src="/_layouts/15/autofill.js"></script> <script src="_layouts/15/sp.core.js"></script> <script> // Run your custom code when the DOM is ready. $(document).ready(function () { // Specify the unique ID of the DOM element where the // picker will render. initializePeoplePicker('peoplePickerDiv'); }); // Render and initialize the client-side People Picker. function initializePeoplePicker(peoplePickerElementId) { // Create a schema to store picker properties, and set the properties. var schema = {}; schema['PrincipalAccountType'] = 'User,DL,SecGroup,SPGroup'; schema['SearchPrincipalSource'] = 15; schema['ResolvePrincipalSource'] = 15; schema['AllowMultipleValues'] = true; schema['MaximumEntitySuggestions'] = 50; schema['Width'] = '280px'; // Render and initialize the picker. // Pass the ID of the DOM element that contains the picker, an array of initial // PickerEntity objects to set the picker value, and a schema that defines // picker properties. this.SPClientPeoplePicker_InitStandaloneControlWrapper(peoplePickerElementId, null, schema); } // Query the picker for user information. function getUserInfo() { // Get the people picker object from the page. var peoplePicker = this.SPClientPeoplePicker.SPClientPeoplePickerDict.peoplePickerDiv_TopSpan; // Get information about all users. var users = peoplePicker.GetAllUserInfo(); var userInfo = ''; for (var i = 0; i < users.length; i++) { var user = users[i]; for (var userProperty in user) { userInfo += userProperty + ': ' + user[userProperty] + '<br>'; } } $('#resolvedUsers').html(userInfo); // Get user keys. var keys = peoplePicker.GetAllUserKeys(); $('#userKeys').html(keys); // Get the first user's ID by using the login name. getUserId(users[0].Key); } // Get the user ID. function getUserId(loginName) { var context = new SP.ClientContext.get_current(); this.user = context.get_web().ensureUser(loginName); context.load(this.user); context.executeQueryAsync( Function.createDelegate(null, ensureUserSuccess), Function.createDelegate(null, onFail) ); } function ensureUserSuccess() { createitem(this.user.get_id()); $('#userId').html(this.user.get_id()); } function onFail(sender, args) { alert('Query failed. Error: ' + args.get_message()); } //Rest API function to create an item in a list function createitem(userId){ $.ajax({ url: _spPageContextInfo.webAbsoluteUrl + "/_api/web/lists/getByTitle('EmpDetails')/Items", type: 'POST', headers: { "Accept": "application/json;odata=verbose", "Content-Type": "application/json;odata=verbose", "X-RequestDigest": $("#__REQUESTDIGEST").val(), "X-HTTP-Method": "POST" }, data: JSON.stringify({ __metadata: { type: "SP.Data.EmpDetailsListItem" }, Title: "Mayuresh Dinkar Joshi", EmployeeNameId: userId, //internalName is 'EmployeeName' but we have to use 'EmployeeNameId' to pass id of a person }), success: function(data) { alert('Item created successfully!'); }, error: function(error) { alert(JSON.stringify(error)); } }); } </script> </head> <body> <div id="peoplePickerDiv"></div> <div> <br/> <input type="button" value="Get User Info and Create Item" onclick="getUserInfo()"></input> <br/> <h1>User info:</h1> <p id="resolvedUsers"></p> <h1>User keys:</h1> <p id="userKeys"></p> <h1>User ID:</h1> <p id="userId"></p> </div> </body> </html>
Step 4: Our custom people picker field will look like as below:
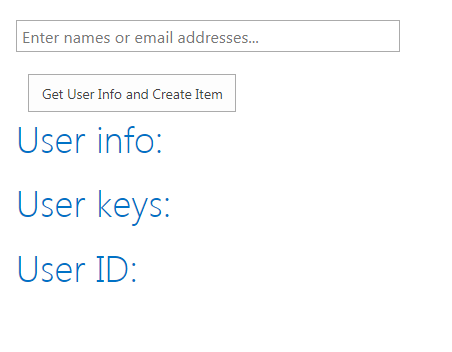
Step 5: When we search for any name and click on button, it will populate that users details and also save the value in our custom list field which we created in step 1. See below screenshots:
Section 2: Get person field value from SharePoint list and set the value to custom people picker field.
Step 1: We have a list named EmpDetails with person or group field as EmployeeName.
Step 2: We will fetch the value for an item with ID=1 and set its value to our custom people picker field.
Step 3: Create a webpart page and add content editor webpart.
Step 4: Add below code in content editor webpart.
Note: I have passed hard-coded URL with item ID 1. I will recommend you to edit the logic accordingly to pass the ID dynamically using text field.
Download below code: Download link
<!--Created by https://www.office365notes.com/2020/10/SharePoint-People-Picker-jQuery-Rest-api.html --> <html> <head> <script src="https://code.jquery.com/jquery-3.5.1.min.js"></script> <script src="/_layouts/15/sp.runtime.js"></script> <script src="/_layouts/15/sp.js"></script> <script src="/_layouts/15/1033/strings.js"></script> <script src="/_layouts/15/clienttemplates.js"></script> <script src="/_layouts/15/clientforms.js"></script> <script src="/_layouts/15/clientpeoplepicker.js"></script> <script src="/_layouts/15/autofill.js"></script> <script src="_layouts/15/sp.core.js"></script> <script> // Run your custom code when the DOM is ready. $(document).ready(function() { // Specify the unique ID of the DOM element where the // picker will render. initializePeoplePicker('peoplePickerDiv'); }); // Render and initialize the client-side People Picker. function initializePeoplePicker(peoplePickerElementId) { // Create a schema to store picker properties, and set the properties. var schema = {}; schema['PrincipalAccountType'] = 'User,DL,SecGroup,SPGroup'; schema['SearchPrincipalSource'] = 15; schema['ResolvePrincipalSource'] = 15; schema['AllowMultipleValues'] = true; schema['MaximumEntitySuggestions'] = 50; schema['Width'] = '280px'; // Render and initialize the picker. // Pass the ID of the DOM element that contains the picker, an array of initial // PickerEntity objects to set the picker value, and a schema that defines // picker properties. this.SPClientPeoplePicker_InitStandaloneControlWrapper(peoplePickerElementId, null, schema); } function readItem() { $.ajax({ url: _spPageContextInfo.webAbsoluteUrl + "/_api/web/lists/getByTitle('EmpDetails')/Items(1)?$select=EmployeeName/EMail&$expand=EmployeeName/Id", type: 'GET', headers: { "accept": "application/json;odata=verbose", }, success: function(data) { console.log(data.d.EmployeeName.EMail); SetAndResolvePeoplePicker(data.d.EmployeeName.EMail); }, error: function(error) { alert(JSON.stringify(error)); } }); } function SetAndResolvePeoplePicker(userAccountName) { var _PeoplePickerEditer = $("#peoplePickerDiv_TopSpan_EditorInput"); userAccountName.split(";#").forEach(function(part) { if (part !== "" && part !== null) { // alert(part); _PeoplePickerEditer.val(part); var _PeoplePickerOject = SPClientPeoplePicker.SPClientPeoplePickerDict["peoplePickerDiv_TopSpan"]; _PeoplePickerOject.AddUnresolvedUserFromEditor(true); } }); } </script> </head> <body> <div id="peoplePickerDiv"></div> <div> <input type="button" value="Set people picker" onclick="readItem()"></input> </div> </body> </html>
Step 5: When we click on a given button, it will call a REST API to fetch the value of person field of list item of ID 1 and set its value to out custom people picker field. We can use this scenario when we need to fetch person fields in our custom edit form.
0 Comments